The How
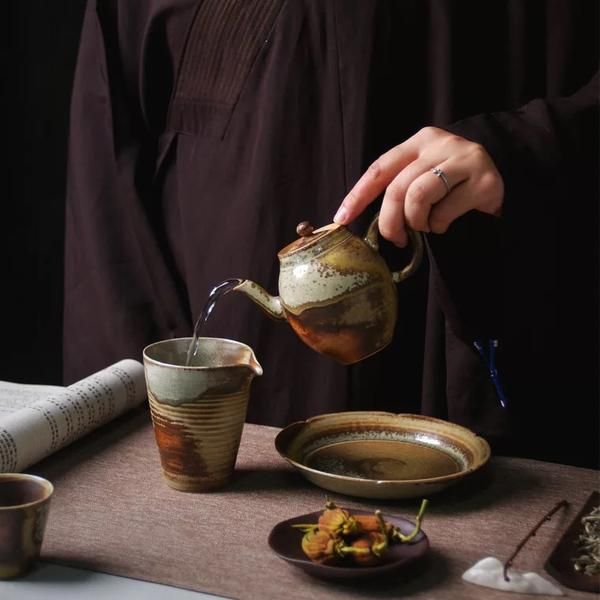
Alright, we have the why (first blog post) now we need the how. The simple answer is Google it! Seriously though, learning how to effectively search for the answers you're looking for is the most primary skill in life that we all need. So becoming competent with Google or a similar search engine is extremely important to quickly becoming a software developer. It can be done with libraries, books and mentors, but if you have that available use it. However, mentorship in a good software engineering practice is still helpful. I intend to describe my practice in this post. I am making the assumption that because you've arrived here you know how to operate a computer in at least a basic way. This assumes you have the following prior knowledge:
- You know at least the high level of what a computer/mouse/keyboard/display is.
- How to get them all connected and powered on.
- How to navigate to a web browser, and how to operate the search bar by typing in a search or a web address in the brower's search bar and initiating the request with the ENTER/RETURN key (or whatever is present in that key location on your keyboard).
- A solid handle of the English language and the capability to look up things that you don't understand about what's present here.
- An understanding that programming computers is about determining what instructions to give a computer to then get a result that you want.
- An understanding that a computer has many, many layers at which you can interact with it and that the portion of the computer doing the actual work takes an immense amount of study to become familiar with.
- Some idea that computers have many different programming languages that they can run if they have right software installed for it.
- Some patience with any errors that you find here. If you're capable of being kind with your words please leave a comment (feature set coming soon) if you believe that there is something incorrect about my claims. I'll update it if it makes sense.
- The rest is up to me to make this useful. So let's dive in. :)
We all have great ideas, ambitions, goals, but not many people get there. It all comes down to execution. For computers, execution can get very tricky. Starting off, it's difficult to get your head around certain concepts. Then, as you start to get an understanding (and see some small success) you might veer away from some solid first principles of life that we should be following. False confidence is the result of those small successes without deep reflection of what's happening. With false confidence we start doing things in a way that is too complicated to be followed by anyone but the original author. The author might make assumptions about prior knowledge and not meet people where they are. Don't fall into that trap, and please always work in a way that others can easily follow. Let me walk you through some of my experiences to highlight the reasoning behind this.
When I was first exposed to programming through one of my introductory engineering courses in college, I could not handle it. I remember taking my shirt off and throwing it against the wall in frustration while my roommate laughed at me not being able to understand loops.
In that situation, I was tasked with writing a short MATLAB script that would simply output a 10x10 grid with either a 1 or 0 in each grid position; depending on whether or not the position was along the diagonal from top left to bottom right. If the position was along the diagonal then it needed to output a 1, and in all other positions it needed to output a 0. The end result looked something like the following in the MATLAB output window when you ran the script:
1 0 0 0 0 0 0 0 0 0
0 1 0 0 0 0 0 0 0 0
0 0 1 0 0 0 0 0 0 0
0 0 0 1 0 0 0 0 0 0
0 0 0 0 1 0 0 0 0 0
0 0 0 0 0 1 0 0 0 0
0 0 0 0 0 0 1 0 0 0
0 0 0 0 0 0 0 1 0 0
0 0 0 0 0 0 0 0 1 0
0 0 0 0 0 0 0 0 0 1
For the life of me I could not tell you how I did this then, and I'm really not a fan of MATLAB nor do I currently have a use case for it. If I did this now I would use Python3 (in depth reading of that language here). The following is the copy of a file named the_how.py saved to my Windows 10 (computer operating system aka computer OS) machine that I have Ubuntu installed on through the Microsoft Store. Ubuntu is a flavor of Linux and itself is a computer operating system. The Microsoft Install of it onto Windows 10 came with Python3 pre-installed. Python3 works on almost any OS and if you don't already have it on your machine, Python3 installation instructions are here (I myself have not gone through this so if there's an issue please comment):
#!/usr/bin/python3
ones_column_position = 0
for row in range(10):
for column in range(10):
if column == ones_column_position:
print("1", end="")
else:
print("0", end="")
if column < 9:
print(" ", end="")
else:
print("")
ones_column_position += 1
This Python3 code does the following:
- It starts with what is called a Shebang line. Which is an identifier for your computer to determine where the python3 interpreter is on your machine. It can be omitted, but omitting it can have side effects which is something we absolutely want to avoid when programming. We want our code to only do what we intend it to do.
- It initializes a variable (programming container for data), named ones_column_position, to the value of 0. It is named ones_column_position to indicate that this variable is keeping track of where the 1's should be as we construct our grid. We're constructing our grid one row at a time and as we're going the column position for the 1's increases by 1 for each row.
- Then we start a for loop (programming construct for repeating certain instructions for a specified number of times) that will iterate 10 times (the Python3 range function is a little bit of a complex one to explain, so take a look at its documentation here). It contains a iteration variable row that will change each time that we run the instructions inside of it. The variable row will start as 0, then it will be 1, then it will be 2, etc... until it reaches the value of 9 and that will be the last time that it runs the instructions contained within it (indicated by levels of indentation). We won't actually be using the row variable, but often times for loop definitions require you to specify an iteration variable and its name helps us understand the loop's purpose. It is guiding our row construction.
- Inside the first for loop we have another one with the same characteristics but it needs a different iteration variable column so that we don't have conflicting names. The computer is just like us in that it needs separation of names in order to easily understand that there's a difference to be accounted for. The column variable will also change from 0-9 as the for loop is processed. This column for loop will be run a total of 10 separate times as the row for loop containing it is dictating that. This for loop is guiding our column construction for each row.
- Inside the column for loop we have the real meat and potatoes of what this code is doing. This is where the computer is making the decisions on when to do things in certain situations, and then also what to do when those situations are met. For our example, the inside of the column loop will be run 100 times. This is because the column loop itself has 10 iterations, and then the row loop is running the column loop 10 times as well. So 10x10 == 100, and that is how we end up creating our grid.
- Now we have statements that are called conditionals. The if statement is a programming construct that says, "if this condition is true, then run the code inside of the if block". It is chained to an else statement which is code that will run if the first conditional is not met. Basically it's if condition do x; else do y. In our situation, for the first conditional, the condition is column == ones_column_position. Which is checking to see if the current value of the variable column is equal to the current value of the variable of ones_column_position. We do this to tell the computer when to print out a 1 (which we're wanting to place on the diagonal) and when to print a 0 (which should be in every other grid position).
- Hoping that the light bulb in your head turns on at this point, and you see that we're just telling the computer to create one grid position at a time. We're giving it detailed instructions to keep performing the same tasks one step at a time. The computer is just very quick to actually perform the tasks you give to it.
- Inside of the conditional is a Python3 function called print() that allows us to output some data to the stdout of the environment that runs the code. In this case it's a terminal window that is running the Ubuntu operating system described earlier in this article. For more information about the print() function check out its documentation here.
- The second conditional is setup to provide formatting so that we are actually creating spacing between grid positions and creating newlines after each 10 positions. Without that additional formatting to create space and newlines the output would just be a straight line like the following:
1000000000010000000000100000000001000000000010000000000100000000001000000000010000000000100000000001
- Now we come to the final line, which is a simple increment reassignment of the ones_column_position variable to replace its value with 1 more than it previously was. So if ones_column_position was 5 before that line, it will be 6 after it. Additionally, we need to place this line in the correct position outside of the column loop, but inside of the row loop so that it gets incremented a total of 10 times; starting at 0 and ending at 9. This allows us to get that cascading output we're looking for with just a single column addition at a time.
Alright, so we've walked through the high level of what our code is doing, and we've described that we have an environment that is capable of running it. To actually run it, you just type the following into your Ubuntu terminal window and you get the following output:
user@machine:~$ python3 the_how.py
1 0 0 0 0 0 0 0 0 0
0 1 0 0 0 0 0 0 0 0
0 0 1 0 0 0 0 0 0 0
0 0 0 1 0 0 0 0 0 0
0 0 0 0 1 0 0 0 0 0
0 0 0 0 0 1 0 0 0 0
0 0 0 0 0 0 1 0 0 0
0 0 0 0 0 0 0 1 0 0
0 0 0 0 0 0 0 0 1 0
0 0 0 0 0 0 0 0 0 1
user@machine:~$ _
So why is any of this significant? Because it's an example of the process of understanding your work well enough to be able to explain it in the simplest of terms. There was the first challenge of needing to do this for course work in order to keep moving forward towards earning my degree. That was extremely painful, but it helped me grow. However, I still didn't understand the bigger picture of what I was doing until years later. I knew that I wanted to be able to create websites or a web presence in order to conduct business online, but having that goal in mind and then getting there without someone expressly looking to help you achieve that goal is a lot. Thankfully, I had many different people provide input to my growth and I am so appreciative of that blessing in my life.
So what is needed is exploration, reflection, understanding, and then paying it forward to those behind you. The most important step that I'm trying to highlight here, is the paying it forward to those behind you. It takes a lot of work to condense and summarize your experience for someone new. That's why it's hard for people to meet you halfway when you're trying to learn. Then think about how hard that would be to scale if you didn't take the time to write it down. The best way to really reach down and pull people up is to document your experience so that you can point to it when having conversations with people. It's also a good way to highlight things that you know if you're looking for new opportunities. So there's just a plethora of valuable things that come from sharing your work with others.
One last important point is this, "Slowly is the fastest way to get to where you want to be." - André De Shields (video clip here).
Another way this was framed for me when I worked at Jackson's Mighty Fine Food and Lucky Lounge; is that if you take the time to do something correctly the first time then you won't have to do it again. So be patient with your work and stand up for sustainable pacing. I really like daily practices that are self contained so that nothing is ever overextended. It avoids burn out so that you can consistently lay a brick on your foundation day after day. Eventually, what you're trying to build will exist and you'll be sitting in the shade of the trees you planted. Don't fall for the tricks of impatient leadership. Those people will lose in the end because they're looking for immediate results instead of progress over the long term that benefits everyone involved. Instead, develop the "Frame of the Veteran" (A concept that was shared to me via Alex Hormozi on Instagram). Simply put, if you don't quit then you won't lose. Eventually you'll win.
That's my how. If it changes I intend to come back to this and update it. The intention is to keep a single source of truth for each conceptual idea so that as it evolves the knowledge storage evolves with it.
Next up, were going to be talking about developing a web presence for yourself! Starting with web domains and some of the interesting use cases that I've seen that are incredibly beneficial for the authors.
Thank you so much for taking the time to read this! Let's keep cracking onward and upward.
Cheers,
DaSeventhHogie